Java Program to print Hallow square pattern
Square frame pattern is designed in accordance to the number of rows user has entered.
In this java program, the user is asked to enter number of rows. It displays a hallow square based on number of rows.
Algorithm:
Step 1: Start.
Step 2: Read number of rows to be printed. Store it in some variable, say n.
Step 3: To iterate through rows, run an outer loop from 1 to n. For that define a for loop.
Step 4: To iterate through columns, run an inner loop from 1 to n. Define a for loop.
Step 5: Inside inner loop print star for the first and last rows and first and last column. Otherwise enter space.
Step 6: Stop.
Source code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
import java.util.Scanner; public class Square { public static void main(String[] args) { System.out.println("\n \n *** www.programmingposts.com *** \n \n"); System.out.println("<< JAVA Program to print hallow Square pattern >> \n \n"); Scanner scanner = new Scanner(System.in); System.out.println("Enter number of rows:"); int rows = scanner.nextInt(); for(int i=1;i<=rows;i++) { for(int j=1;j<=rows;j++) { if(i==1 || i==rows || j==1 || j==rows) { System.out.print("* "); } else { System.out.print(" "); } } System.out.println(); } } } |
Explanation:
for(int i=1;i<=rows;i++) – To iterate through rows run an outer loop from 1 to rows.
for(int j=1;j<=rows;j++) – To iterate through columns, run an inner loop from 1 to rows.
if(i==1 || i==rows || j==1 || j==rows) – Inside inner loop print star for first and last row or for first and last column.
else print space.
Output:
C:\Users\santo\Desktop\new>javac Square.java
C:\Users\santo\Desktop\new>java Square
*** www.programmingposts.com ***
<< JAVA Program to print hallow square pattern >>
Enter number of rows:
6
* * * * * *
* *
* *
* *
* *
* * * * * *
Screenshot of Output:
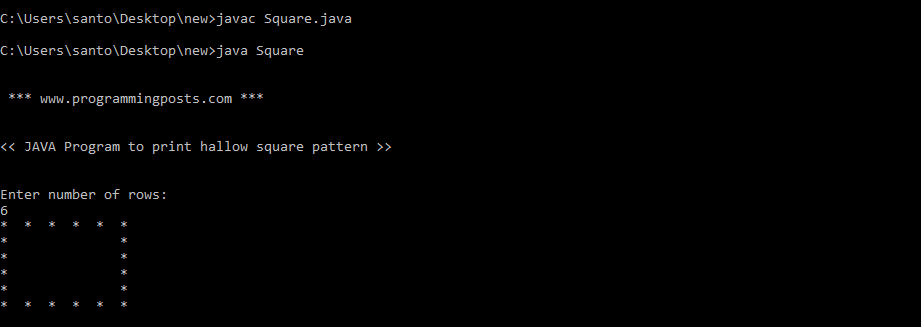