DELETING A ROW DYNAMICALLY IN A GRID VIEW IN ASP.NET
In this post we will see the RowDeleting event in Grid View in Asp.net. That is to delete the row in a grid view dynamically.
For this take a grid view in a form default.aspx and add columns to the grid view. And add one extra column, Delete in a Command Field.
For this take a grid view in a form default.aspx and add columns to the grid view. And add one extra column, Delete in a Command Field.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <table width="100%"> <tr> <td> </td> <td> <br /> <asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" BackColor="White" BorderColor="#CC9966" BorderStyle="None" BorderWidth="1px" CellPadding="4" OnRowDeleting="GridView1_RowDeleting"> <Columns> <asp:CommandField ShowDeleteButton="True" /> <asp:BoundField DataField="Emp_Id" HeaderText="EMP ID" /> <asp:BoundField DataField="Emp_Name" HeaderText="EMP NAME" /> <asp:BoundField DataField="Location" HeaderText="Location" /> </Columns> <FooterStyle BackColor="#FFFFCC" ForeColor="#330099" /> <HeaderStyle BackColor="#990000" Font-Bold="True" ForeColor="#FFFFCC" /> <PagerStyle BackColor="#FFFFCC" ForeColor="#330099" HorizontalAlign="Center" /> <RowStyle BackColor="White" ForeColor="#330099" /> <SelectedRowStyle BackColor="#FFCC66" Font-Bold="True" ForeColor="#663399" /> <SortedAscendingCellStyle BackColor="#FEFCEB" /> <SortedAscendingHeaderStyle BackColor="#AF0101" /> <SortedDescendingCellStyle BackColor="#F6F0C0" /> <SortedDescendingHeaderStyle BackColor="#7E0000" /> </asp:GridView> <br /> <asp:Label ID="lblMessage" runat="server" Text="Message"></asp:Label> </td> </tr> </table> <br /> </div> </form> </body> </html> |
In the code behind file the c# code is like this :
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
using System; using System.Data; using System.Data.SqlClient; using System.Configuration; using System.Web.UI.WebControls; public partial class _Default : System.Web.UI.Page { SqlConnection con = new SqlConnection("Data Source=LocalHost;Integrated security=true;Initial Catalog=sameer"); SqlCommand cmd; SqlDataAdapter da; DataTable table = new DataTable(); protected void Page_Load(object sender, EventArgs e) { BindGridData(); } public void BindGridData() //function to bind data to gridview { cmd = new SqlCommand("SELECT * FROM EMP_DETAILS", con); da = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); da.Fill(ds); GridView1.DataSource = ds; GridView1.DataBind(); } protected void GridView1_RowDeleting(object sender, System.Web.UI.WebControls.GridViewDeleteEventArgs e) { TableCell Emp_Id = GridView1.Rows[e.RowIndex].Cells[1]; cmd = new SqlCommand("DELETE FROM EMP_DETAILS WHERE EMP_ID="+Emp_Id.Text,con); con.Open(); cmd.ExecuteNonQuery(); con.Close(); BindGridData(); lblMessage.Text = "Row deleted successfully"; } } |
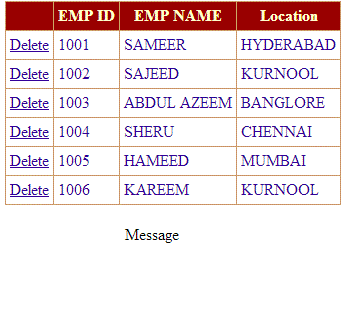