C PROGRAM TO COUNT AND PRINT VOWELS IN A GIVEN STRING
/*** THIS C PROGRAM TAKES A LINE OF STRING AS INPUT AND PRINTS THE VOWELS AND THE COUNT OF VOWELS OCCURRED IN IT ***/
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include<stdio.h> #include<conio.h> #define MAX 100 //limiting the maximum size of string #define SIZE 5 //we have 5 vowels a,e,i,o,u main() { int i,j; char str[MAX],vowelcount[SIZE]; char vowels[SIZE]={'a','e','i','o','u'}; //clrscr(); printf("\nPROGRAM TO COUNT VOWELS\n"); printf("\nEnter the String:"); //scanf("%s",&str); //this allows only a string(single word) gets(str); //this allows a line as string for(i=0;i<5;i++) { vowelcount[i]=0; } for(i=0;str[i]!='\0';i++) { for(j=0;j<SIZE;j++) { if((str[i]==vowels[j])||(str[i]==vowels[j]-32)) { vowelcount[j]=vowelcount[j]++; } } } for(i=0;i<SIZE;i++) //printing vowels and its count { printf("\n %c occured:%d times",vowels[i],vowelcount[i]); } getch(); } |
OUTPUT:
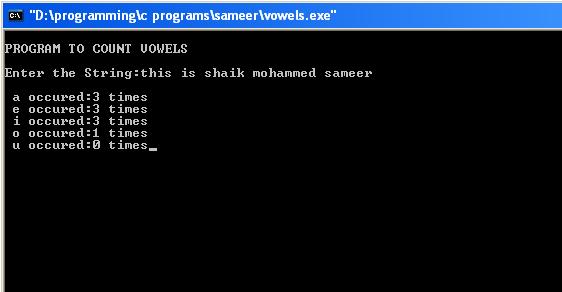